mirror of
https://codeberg.org/forgejo/forgejo.git
synced 2024-11-01 14:59:06 +00:00
releated to #21820 - Split `Size` in repository table as two new colunms, one is `GitSize` for git size, the other is `LFSSize` for lfs data. still store full size in `Size` colunm. - Show full size on ui, but show each of them by a `title`; example: 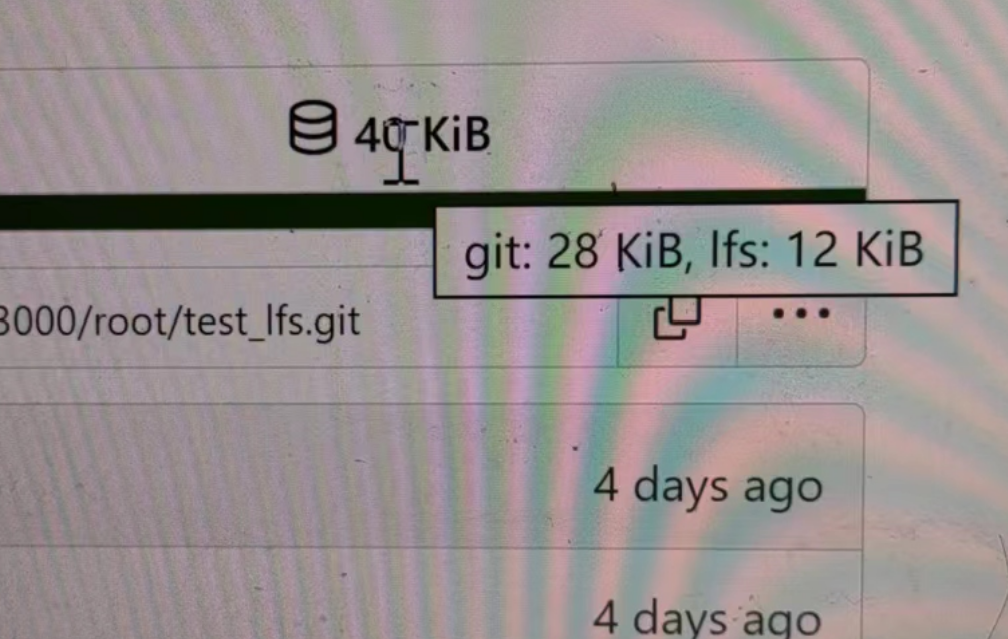 - Return full size in api response. --------- Signed-off-by: a1012112796 <1012112796@qq.com> Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com> Co-authored-by: silverwind <me@silverwind.io> Co-authored-by: DmitryFrolovTri <23313323+DmitryFrolovTri@users.noreply.github.com> Co-authored-by: Giteabot <teabot@gitea.io>
182 lines
4.9 KiB
Go
182 lines
4.9 KiB
Go
// Copyright 2021 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package explore
|
|
|
|
import (
|
|
"fmt"
|
|
"net/http"
|
|
|
|
"code.gitea.io/gitea/models/db"
|
|
repo_model "code.gitea.io/gitea/models/repo"
|
|
"code.gitea.io/gitea/modules/base"
|
|
"code.gitea.io/gitea/modules/context"
|
|
"code.gitea.io/gitea/modules/log"
|
|
"code.gitea.io/gitea/modules/setting"
|
|
"code.gitea.io/gitea/modules/sitemap"
|
|
)
|
|
|
|
const (
|
|
// tplExploreRepos explore repositories page template
|
|
tplExploreRepos base.TplName = "explore/repos"
|
|
relevantReposOnlyParam string = "only_show_relevant"
|
|
)
|
|
|
|
// RepoSearchOptions when calling search repositories
|
|
type RepoSearchOptions struct {
|
|
OwnerID int64
|
|
Private bool
|
|
Restricted bool
|
|
PageSize int
|
|
OnlyShowRelevant bool
|
|
TplName base.TplName
|
|
}
|
|
|
|
// RenderRepoSearch render repositories search page
|
|
// This function is also used to render the Admin Repository Management page.
|
|
func RenderRepoSearch(ctx *context.Context, opts *RepoSearchOptions) {
|
|
// Sitemap index for sitemap paths
|
|
page := int(ctx.ParamsInt64("idx"))
|
|
isSitemap := ctx.Params("idx") != ""
|
|
if page <= 1 {
|
|
page = ctx.FormInt("page")
|
|
}
|
|
|
|
if page <= 0 {
|
|
page = 1
|
|
}
|
|
|
|
if isSitemap {
|
|
opts.PageSize = setting.UI.SitemapPagingNum
|
|
}
|
|
|
|
var (
|
|
repos []*repo_model.Repository
|
|
count int64
|
|
err error
|
|
orderBy db.SearchOrderBy
|
|
)
|
|
|
|
ctx.Data["SortType"] = ctx.FormString("sort")
|
|
switch ctx.FormString("sort") {
|
|
case "newest":
|
|
orderBy = db.SearchOrderByNewest
|
|
case "oldest":
|
|
orderBy = db.SearchOrderByOldest
|
|
case "leastupdate":
|
|
orderBy = db.SearchOrderByLeastUpdated
|
|
case "reversealphabetically":
|
|
orderBy = db.SearchOrderByAlphabeticallyReverse
|
|
case "alphabetically":
|
|
orderBy = db.SearchOrderByAlphabetically
|
|
case "reversesize":
|
|
orderBy = db.SearchOrderBySizeReverse
|
|
case "size":
|
|
orderBy = db.SearchOrderBySize
|
|
case "reversegitsize":
|
|
orderBy = db.SearchOrderByGitSizeReverse
|
|
case "gitsize":
|
|
orderBy = db.SearchOrderByGitSize
|
|
case "reverselfssize":
|
|
orderBy = db.SearchOrderByLFSSizeReverse
|
|
case "lfssize":
|
|
orderBy = db.SearchOrderByLFSSize
|
|
case "moststars":
|
|
orderBy = db.SearchOrderByStarsReverse
|
|
case "feweststars":
|
|
orderBy = db.SearchOrderByStars
|
|
case "mostforks":
|
|
orderBy = db.SearchOrderByForksReverse
|
|
case "fewestforks":
|
|
orderBy = db.SearchOrderByForks
|
|
default:
|
|
ctx.Data["SortType"] = "recentupdate"
|
|
orderBy = db.SearchOrderByRecentUpdated
|
|
}
|
|
|
|
keyword := ctx.FormTrim("q")
|
|
|
|
ctx.Data["OnlyShowRelevant"] = opts.OnlyShowRelevant
|
|
|
|
topicOnly := ctx.FormBool("topic")
|
|
ctx.Data["TopicOnly"] = topicOnly
|
|
|
|
language := ctx.FormTrim("language")
|
|
ctx.Data["Language"] = language
|
|
|
|
repos, count, err = repo_model.SearchRepository(ctx, &repo_model.SearchRepoOptions{
|
|
ListOptions: db.ListOptions{
|
|
Page: page,
|
|
PageSize: opts.PageSize,
|
|
},
|
|
Actor: ctx.Doer,
|
|
OrderBy: orderBy,
|
|
Private: opts.Private,
|
|
Keyword: keyword,
|
|
OwnerID: opts.OwnerID,
|
|
AllPublic: true,
|
|
AllLimited: true,
|
|
TopicOnly: topicOnly,
|
|
Language: language,
|
|
IncludeDescription: setting.UI.SearchRepoDescription,
|
|
OnlyShowRelevant: opts.OnlyShowRelevant,
|
|
})
|
|
if err != nil {
|
|
ctx.ServerError("SearchRepository", err)
|
|
return
|
|
}
|
|
if isSitemap {
|
|
m := sitemap.NewSitemap()
|
|
for _, item := range repos {
|
|
m.Add(sitemap.URL{URL: item.HTMLURL(), LastMod: item.UpdatedUnix.AsTimePtr()})
|
|
}
|
|
ctx.Resp.Header().Set("Content-Type", "text/xml")
|
|
if _, err := m.WriteTo(ctx.Resp); err != nil {
|
|
log.Error("Failed writing sitemap: %v", err)
|
|
}
|
|
return
|
|
}
|
|
|
|
ctx.Data["Keyword"] = keyword
|
|
ctx.Data["Total"] = count
|
|
ctx.Data["Repos"] = repos
|
|
ctx.Data["IsRepoIndexerEnabled"] = setting.Indexer.RepoIndexerEnabled
|
|
|
|
pager := context.NewPagination(int(count), opts.PageSize, page, 5)
|
|
pager.SetDefaultParams(ctx)
|
|
pager.AddParam(ctx, "topic", "TopicOnly")
|
|
pager.AddParam(ctx, "language", "Language")
|
|
pager.AddParamString(relevantReposOnlyParam, fmt.Sprint(opts.OnlyShowRelevant))
|
|
ctx.Data["Page"] = pager
|
|
|
|
ctx.HTML(http.StatusOK, opts.TplName)
|
|
}
|
|
|
|
// Repos render explore repositories page
|
|
func Repos(ctx *context.Context) {
|
|
ctx.Data["UsersIsDisabled"] = setting.Service.Explore.DisableUsersPage
|
|
ctx.Data["Title"] = ctx.Tr("explore")
|
|
ctx.Data["PageIsExplore"] = true
|
|
ctx.Data["PageIsExploreRepositories"] = true
|
|
ctx.Data["IsRepoIndexerEnabled"] = setting.Indexer.RepoIndexerEnabled
|
|
|
|
var ownerID int64
|
|
if ctx.Doer != nil && !ctx.Doer.IsAdmin {
|
|
ownerID = ctx.Doer.ID
|
|
}
|
|
|
|
onlyShowRelevant := setting.UI.OnlyShowRelevantRepos
|
|
|
|
_ = ctx.Req.ParseForm() // parse the form first, to prepare the ctx.Req.Form field
|
|
if len(ctx.Req.Form[relevantReposOnlyParam]) != 0 {
|
|
onlyShowRelevant = ctx.FormBool(relevantReposOnlyParam)
|
|
}
|
|
|
|
RenderRepoSearch(ctx, &RepoSearchOptions{
|
|
PageSize: setting.UI.ExplorePagingNum,
|
|
OwnerID: ownerID,
|
|
Private: ctx.Doer != nil,
|
|
TplName: tplExploreRepos,
|
|
OnlyShowRelevant: onlyShowRelevant,
|
|
})
|
|
}
|