mirror of
https://codeberg.org/forgejo/forgejo.git
synced 2024-06-10 09:29:32 +00:00
Before there was a "graceful function": RunWithShutdownFns, it's mainly for some modules which doesn't support context. The old queue system doesn't work well with context, so the old queues need it. After the queue refactoring, the new queue works with context well, so, use Golang context as much as possible, the `RunWithShutdownFns` could be removed (replaced by RunWithCancel for context cancel mechanism), the related code could be simplified. This PR also fixes some legacy queue-init problems, eg: * typo : archiver: "unable to create codes indexer queue" => "unable to create repo-archive queue" * no nil check for failed queues, which causes unfriendly panic After this PR, many goroutines could have better display name: 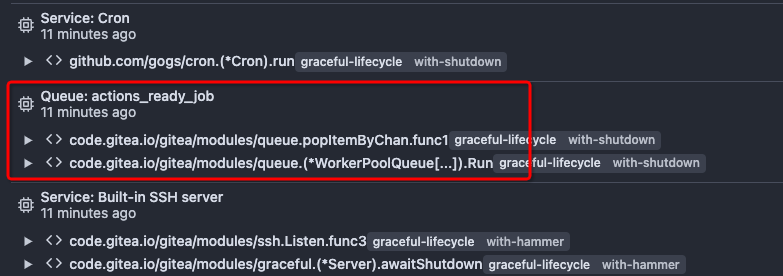 
71 lines
1.8 KiB
Go
71 lines
1.8 KiB
Go
// Copyright 2022 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package mirror
|
|
|
|
import (
|
|
"code.gitea.io/gitea/modules/graceful"
|
|
"code.gitea.io/gitea/modules/log"
|
|
"code.gitea.io/gitea/modules/queue"
|
|
"code.gitea.io/gitea/modules/setting"
|
|
)
|
|
|
|
var mirrorQueue *queue.WorkerPoolQueue[*SyncRequest]
|
|
|
|
// SyncType type of sync request
|
|
type SyncType int
|
|
|
|
const (
|
|
// PullMirrorType for pull mirrors
|
|
PullMirrorType SyncType = iota
|
|
// PushMirrorType for push mirrors
|
|
PushMirrorType
|
|
)
|
|
|
|
// SyncRequest for the mirror queue
|
|
type SyncRequest struct {
|
|
Type SyncType
|
|
ReferenceID int64 // RepoID for pull mirror, MirrorID for push mirror
|
|
}
|
|
|
|
// StartSyncMirrors starts a go routine to sync the mirrors
|
|
func StartSyncMirrors(queueHandle func(data ...*SyncRequest) []*SyncRequest) {
|
|
if !setting.Mirror.Enabled {
|
|
return
|
|
}
|
|
mirrorQueue = queue.CreateUniqueQueue(graceful.GetManager().ShutdownContext(), "mirror", queueHandle)
|
|
if mirrorQueue == nil {
|
|
log.Fatal("Unable to create mirror queue")
|
|
}
|
|
go graceful.GetManager().RunWithCancel(mirrorQueue)
|
|
}
|
|
|
|
// AddPullMirrorToQueue adds repoID to mirror queue
|
|
func AddPullMirrorToQueue(repoID int64) {
|
|
addMirrorToQueue(PullMirrorType, repoID)
|
|
}
|
|
|
|
// AddPushMirrorToQueue adds the push mirror to the queue
|
|
func AddPushMirrorToQueue(mirrorID int64) {
|
|
addMirrorToQueue(PushMirrorType, mirrorID)
|
|
}
|
|
|
|
func addMirrorToQueue(syncType SyncType, referenceID int64) {
|
|
if !setting.Mirror.Enabled {
|
|
return
|
|
}
|
|
go func() {
|
|
if err := PushToQueue(syncType, referenceID); err != nil {
|
|
log.Error("Unable to push sync request for to the queue for pull mirror repo[%d]. Error: %v", referenceID, err)
|
|
}
|
|
}()
|
|
}
|
|
|
|
// PushToQueue adds the sync request to the queue
|
|
func PushToQueue(mirrorType SyncType, referenceID int64) error {
|
|
return mirrorQueue.Push(&SyncRequest{
|
|
Type: mirrorType,
|
|
ReferenceID: referenceID,
|
|
})
|
|
}
|