2022-06-03 21:38:26 +00:00
import $ from 'jquery' ;
let ariaIdCounter = 0 ;
function generateAriaId ( ) {
return ` _aria_auto_id_ ${ ariaIdCounter ++ } ` ;
}
// make the item has role=option, and add an id if there wasn't one yet.
function prepareMenuItem ( $item ) {
if ( ! $item . attr ( 'id' ) ) $item . attr ( 'id' , generateAriaId ( ) ) ;
$item . attr ( { 'role' : 'menuitem' , 'tabindex' : '-1' } ) ;
$item . find ( 'a' ) . attr ( 'tabindex' , '-1' ) ; // as above, the elements inside the dropdown menu item should not be focusable, the focus should always be on the dropdown primary element.
}
// when the menu items are loaded from AJAX requests, the items are created dynamically
const defaultCreateDynamicMenu = $ . fn . dropdown . settings . templates . menu ;
$ . fn . dropdown . settings . templates . menu = function ( response , fields , preserveHTML , className ) {
const ret = defaultCreateDynamicMenu ( response , fields , preserveHTML , className ) ;
const $wrapper = $ ( '<div>' ) . append ( ret ) ;
const $items = $wrapper . find ( '> .item' ) ;
$items . each ( ( _ , item ) => {
prepareMenuItem ( $ ( item ) ) ;
} ) ;
return $wrapper . html ( ) ;
} ;
function attachOneDropdownAria ( $dropdown ) {
if ( $dropdown . attr ( 'data-aria-attached' ) ) return ;
$dropdown . attr ( 'data-aria-attached' , 1 ) ;
const $textSearch = $dropdown . find ( 'input.search' ) . eq ( 0 ) ;
const $focusable = $textSearch . length ? $textSearch : $dropdown ; // see comment below
if ( ! $focusable . length ) return ;
// prepare menu list
const $menu = $dropdown . find ( '> .menu' ) ;
if ( ! $menu . attr ( 'id' ) ) $menu . attr ( 'id' , generateAriaId ( ) ) ;
// dropdown has 2 different focusing behaviors
// * with search input: the input is focused, and it works perfectly with aria-activedescendant pointing another sibling element.
// * without search input (but the readonly text), the dropdown itself is focused. then the aria-activedescendant points to the element inside dropdown
// expected user interactions for dropdown with aria support:
// * user can use Tab to focus in the dropdown, then the dropdown menu (list) will be shown
// * user presses Tab on the focused dropdown to move focus to next sibling focusable element (but not the menu item)
// * user can use arrow key Up/Down to navigate between menu items
// * when user presses Enter:
// - if the menu item is clickable (eg: <a>), then trigger the click event
// - otherwise, the dropdown control (low-level code) handles the Enter event, hides the dropdown menu
// TODO: multiple selection is not supported yet.
$focusable . attr ( {
'role' : 'menu' ,
'aria-haspopup' : 'menu' ,
'aria-controls' : $menu . attr ( 'id' ) ,
'aria-expanded' : 'false' ,
} ) ;
if ( $dropdown . attr ( 'data-content' ) && ! $dropdown . attr ( 'aria-label' ) ) {
$dropdown . attr ( 'aria-label' , $dropdown . attr ( 'data-content' ) ) ;
}
$menu . find ( '> .item' ) . each ( ( _ , item ) => {
prepareMenuItem ( $ ( item ) ) ;
} ) ;
// update aria attributes according to current active/selected item
const refreshAria = ( ) => {
const isMenuVisible = ! $menu . is ( '.hidden' ) && ! $menu . is ( '.animating.out' ) ;
$focusable . attr ( 'aria-expanded' , isMenuVisible ? 'true' : 'false' ) ;
let $active = $menu . find ( '> .item.active' ) ;
if ( ! $active . length ) $active = $menu . find ( '> .item.selected' ) ; // it's strange that we need this fallback at the moment
// if there is an active item, use its id. if no active item, then the empty string is set
$focusable . attr ( 'aria-activedescendant' , $active . attr ( 'id' ) ) ;
} ;
$dropdown . on ( 'keydown' , ( e ) => {
// here it must use keydown event before dropdown's keyup handler, otherwise there is no Enter event in our keyup handler
if ( e . key === 'Enter' ) {
Make issue meta dropdown support Enter, confirm before reloading (#23014) (#23102)
Backport #23014
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
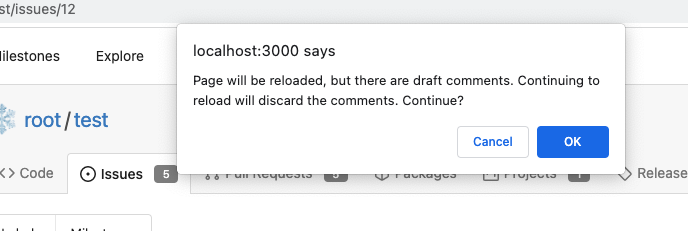
</details>
Co-authored-by: wxiaoguang <wxiaoguang@gmail.com>
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 09:40:36 +00:00
let $item = $dropdown . dropdown ( 'get item' , $dropdown . dropdown ( 'get value' ) ) ;
if ( ! $item ) $item = $menu . find ( '> .item.selected' ) ; // when dropdown filters items by input, there is no "value", so query the "selected" item
2022-06-03 21:38:26 +00:00
// if the selected item is clickable, then trigger the click event. in the future there could be a special CSS class for it.
if ( $item && $item . is ( 'a' ) ) $item [ 0 ] . click ( ) ;
}
} ) ;
// use setTimeout to run the refreshAria in next tick (to make sure the Fomantic UI code has finished its work)
const deferredRefreshAria = ( ) => { setTimeout ( refreshAria , 0 ) } ; // do not return any value, jQuery has return-value related behaviors.
$focusable . on ( 'focus' , deferredRefreshAria ) ;
$focusable . on ( 'mouseup' , deferredRefreshAria ) ;
$focusable . on ( 'blur' , deferredRefreshAria ) ;
$dropdown . on ( 'keyup' , ( e ) => { if ( e . key . startsWith ( 'Arrow' ) ) deferredRefreshAria ( ) ; } ) ;
}
export function attachDropdownAria ( $dropdowns ) {
$dropdowns . each ( ( _ , e ) => attachOneDropdownAria ( $ ( e ) ) ) ;
}
2023-01-25 15:52:10 +00:00
export function attachCheckboxAria ( $checkboxes ) {
$checkboxes . checkbox ( ) ;
// Fomantic UI checkbox needs to be something like: <div class="ui checkbox"><label /><input /></div>
// It doesn't work well with <label><input />...</label>
// To make it work with aria, the "id"/"for" attributes are necessary, so add them automatically if missing.
// In the future, refactor to use native checkbox directly, then this patch could be removed.
for ( const el of $checkboxes ) {
const label = el . querySelector ( 'label' ) ;
const input = el . querySelector ( 'input' ) ;
if ( ! label || ! input || input . getAttribute ( 'id' ) ) continue ;
const id = generateAriaId ( ) ;
input . setAttribute ( 'id' , id ) ;
label . setAttribute ( 'for' , id ) ;
}
}